In this blog post, we are going to learn to resolve the conflicts with merge requests or pull requests in Git codebase.
Let's assume we have two branches and trying to merging code from develop branch to master branch. 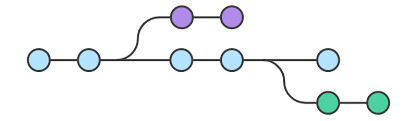 |
Git merge conflicts | Atlassian |
Source branch: develop
Destination branch: master
Step1: Open Git bash and check out the latest source branch code in local directory. e.g. git checkout develop This Git command will pull the latest code from develop branch in the local repo and this will change the current working branch to develop in Git Bash.
Step2: Now we need to pull the code of the destination branch into the source branch. Hence in our case, we will run Git command git pull origin master. This command will pull the code from the destination and match it with the source. This will also show you a list of all the files where conflict needs to be resolve to successfully merge.
Example:
Auto-merging src/header.java
CONFLICT (content): Merge conflict in src/header.java
Automatic merge failed; fix conflicts and then commit the result.
Step3: Now open your developer IDE and look for above mentioned file [src/header.java], and see where the conflict is. IDE by default also gives some hint to accept the source changes or destination changes, you may just click on that option and keep and go only with one change. If you think that option is not sufficed your need and you have to manually make some changes in code then go ahead and make the required changes and save the changes.
Step4: Since we have fixed the code changes, now it is time to check-in the code into the source branch. To check-in the code uses the below commands in Git one by one.
git add <file name> or .(dot) if we wants to add all the files.
git commit -m "commit message"
git push origin develop
Step5: Now go to merge request and see, the conflicts will be get resolved. And now we could merge the code.
Reference:
Bitbucket merge conflict